How to Check if a String Contains a Substring in Bash
Updated on
•6 min read
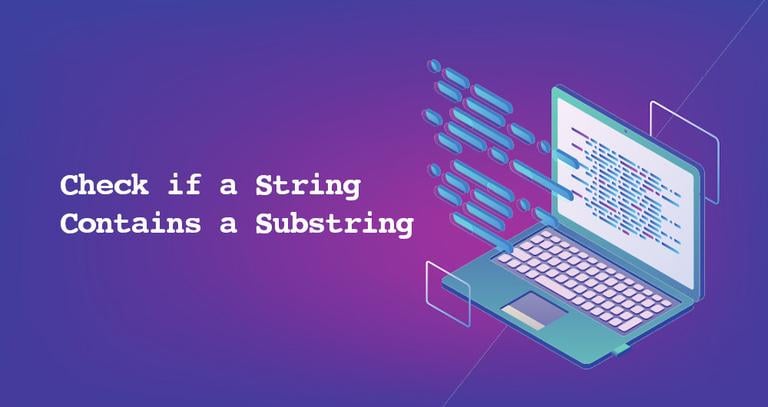
One of the most common operations when working with strings in Bash is to determine whether or not a string contains another string.
In this article, we will show you several ways to check if a string contains a substring.
Using Wildcards
The easiest approach is to surround the substring with asterisk wildcard symbols (asterisk) *
and compare
it with the string. Wildcard is a symbol used to represent zero, one or more characters.
If the test returns true
, the substring is contained in the string.
In the example below we are using the if statement
and the equality operator (==
) to check whether the substring SUB
is found within the string STR
:
#!/bin/bash
STR='GNU/Linux is an operating system'
SUB='Linux'
if [[ "$STR" == *"$SUB"* ]]; then
echo "It's there."
fi
When executed the script will output:
It's there.
Using the case operator
Instead of using the if statement you can also use the case statement to check whether or not a string includes another string.
#!/bin/bash
STR='GNU/Linux is an operating system'
SUB='Linux'
case $STR in
*"$SUB"*)
echo -n "It's there."
;;
esac
Using Regex Operator
Another option to determine whether a specified substring occurs within a string is to use the regex operator =~
. When this operator is used, the right string is considered as a regular expression.
The period followed by an asterisk .*
matches zero or more occurrences any character except a newline character.
#!/bin/bash
STR='GNU/Linux is an operating system'
SUB='Linux'
if [[ "$STR" =~ .*"$SUB".* ]]; then
echo "It's there."
fi
The script will echo the following:
It's there.
Using Grep
The grep command can also be used to find strings in another string.
In the following example, we are passing the string $STR
as an input to grep and checking if the string $SUB
is found within the input string. The command will return true
or false
as appropriate.
#!/bin/bash
STR='GNU/Linux is an operating system'
SUB='Linux'
if grep -q "$SUB" <<< "$STR"; then
echo "It's there"
fi
The -q
option tells grep to be quiet, to omit the output.
Conclusion
Checking if a string contains a substring is one of the most basic and frequently used operations in Bash scripting.
After reading this tutorial, you should have a good understanding of how to test whether a string includes another string. You can also use other commands like awk
or sed
for testing.
If you have any questions or feedback, feel free to leave a comment.